Technical Seo Configurator For Laravel By Honeystone
Last updated on
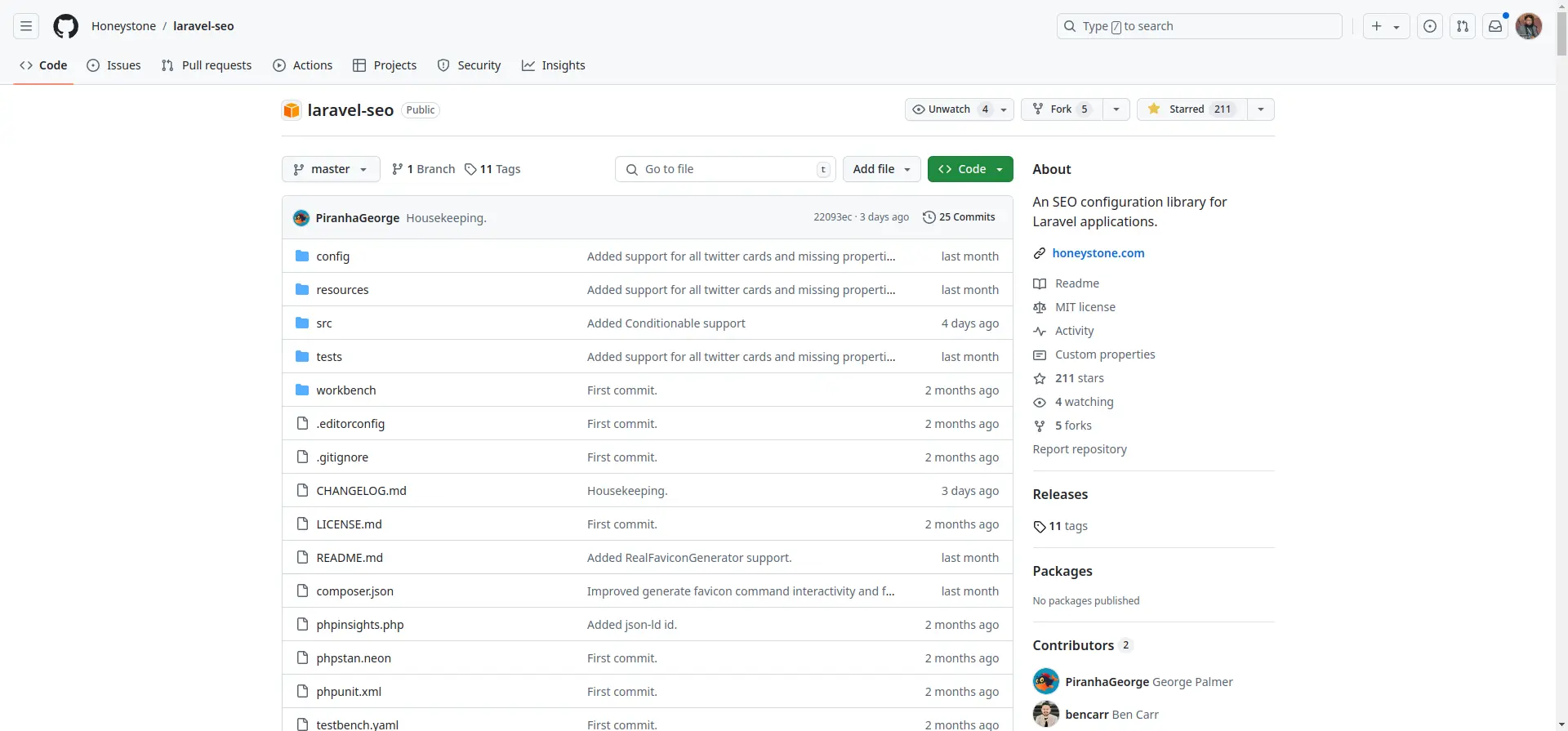
Technical SEO Configurator for Laravel by honeystone
Improve Your Laravel Ecommerce Store Technical Seo Using butschster/laravelmetatags could be a good alternative for managing Technical SEO in your Laravel project.
The Honeystone SEO package makes configuring SEO metadata from anywhere within your Laravel application a breeze.
Included are metadata generators for general metadata, X (Formally Twitter) Cards, Open Graph, JSON-LD Schema, and Favicons (generated using RealFaviconGenerator).
This package was designed with extensibility in mind, so your own custom metadata generators can also be added with ease.
Installation
1composer require honeystone/laravel-seo
Publish the configuration file with:
1php artisan vendor:publish --tag=honeystone-seo-config
Usage
The package provides a helper function, seo()
, and some Blade directives, @metadata
and @openGraphPrefix
. You
can also typehint the Honeystone\Seo\MetadataDirector
if you prefer to use dependency injection.
Setting metadata is a simple as chaining methods:
1seo()2 ->title('A fantastic blog post', 'My Awesome Website!')3 ->description('Theres really a lot of great stuff in here...')4 ->images(5 'https://mywebsite.com/images/blog-1/cover-image.webp',6 'https://mywebsite.com/images/blog-1/another-image.webp',7 );
Once you've set your metadata, you can render it using:
1seo()->generate();
Alternatively, you can also use the @metadata
Blade directive.
The rendered result will look something like this:
1<title>A fantastic blog post - My Awesome Website!</title> 2<meta name="description" content="Theres really a lot of great stuff in here..."> 3<link rel="canonical" href="https://mywebsite.com/blog/a-fantastic-blog-post"> 4 5<!-- Twitter Cards --> 6<meta name="twitter:card" content="summary_large_image"> 7<meta name="twitter:title" content="A fantastic blog post"> 8<meta name="twitter:description" content="Theres really a lot of great stuff in here..."> 9<meta name="twitter:image" content="https://mywebsite.com/images/blog-1/cover-image.webp">10 11<!-- Open Graph -->12<meta property="og:type" content="website">13<meta property="og:title" content="A fantastic blog post">14<meta property="og:description" content="Theres really a lot of great stuff in here...">15<meta property="og:image" content="https://mywebsite.com/images/blog-1/cover-image.webp">16<meta property="og:image" content="https://mywebsite.com/images/blog-1/another-image.webp">17<meta property="og:url" content="https://mywebsite.com/blog/a-fantastic-blog-post">18 19<!-- JSON-LD -->20<script type="application/ld+json">21 {22 "@context": "https://schema.org",23 "@type": "WebPage",24 "name": "A fantastic blog post",25 "description": "Theres really a lot of great stuff in here...",26 "image": [27 "https://mywebsite.com/images/blog-1/cover-image.webp",28 "https://mywebsite.com/images/blog-1/another-image.webp"29 ],30 "url": "https://mywebsite.com"31 }32</script>
Default methods
Values provided to default methods will automatically propagate to all configured metadata generators.
The following default methods are available:
1seo() 2 ->locale('en_GB') 3 ->title('A fantastic blog post', template: '🔥🔥 {title} 🔥🔥') 4 ->description('Theres really a lot of great stuff in here...') 5 ->keywords('foo', 'bar', 'baz') 6 ->url('https://mywebsite.com/blog/a-fantastic-blog-post') //defaults to the current url 7 ->canonical('https://mywebsite.com/blog/a-fantastic-blog-post') //by default url and canonical are in sync, see config 8 ->canonicalEnabled(true) //enabled by default, see config 9 ->images(10 'https://mywebsite.com/images/blog-1/cover-image.webp',11 'https://mywebsite.com/images/blog-1/another-image.webp',12 )13 ->robots('🤖', '🤖', '🤖');
The full baseline looks like this:
1<title>🔥🔥 A fantastic blog post 🔥🔥</title> 2<meta name="description" content="Theres really a lot of great stuff in here..."> 3<meta name="keywords" content="foo,bar,baz"> 4<link rel="canonical" href="https://mywebsite.com/blog/a-fantastic-blog-post"> 5<meta name="robots" content="🤖,🤖,🤖"> 6 7<!-- Twitter Cards --> 8<meta name="twitter:card" content="summary_large_image"> 9<meta name="twitter:title" content="A fantastic blog post">10<meta name="twitter:description" content="Theres really a lot of great stuff in here...">11<meta name="twitter:image" content="https://mywebsite.com/images/blog-1/cover-image.webp">12 13<!-- Open Graph -->14<meta property="og:site_name" content="My Website">15<meta property="og:title" content="A fantastic blog post">16<meta property="og:description" content="Theres really a lot of great stuff in here...">17<meta property="og:image" content="https://mywebsite.com/images/blog-1/cover-image.webp">18<meta property="og:image" content="https://mywebsite.com/images/blog-1/another-image.webp">19<meta property="og:url" content="https://mywebsite.com/blog/a-fantastic-blog-post">20<meta property="og:locale" content="en_GB">21 22<!-- JSON-LD -->23<script type="application/ld+json">24 {25 "@context": "https://schema.org",26 "@type": "WebPage",27 "name": "A fantastic blog post",28 "description": "Theres really a lot of great stuff in here...",29 "image": [30 "https://mywebsite.com/images/blog-1/cover-image.webp",31 "https://mywebsite.com/images/blog-1/another-image.webp"32 ],33 "url": "https://mywebsite.com/blog/a-fantastic-blog-post"34 }35</script>
For your homepage you'll probably want to disable the title template:
1seo()->title('My Awesome Website!', template: false);
Meta methods
The meta methods are provided by the Honeystone\Seo\Generators\MetaGenerator
class.
Here's the full list:
1seo()2 ->metaTitle('A fantastic blog post')3 ->metaTitleTemplate('🔥🔥 {title} 🔥🔥')4 ->metaDescription('Theres really a lot of great stuff in here...')5 ->metaKeywords('foo', 'bar', 'baz')6 ->metaCanonical('https://mywebsite.com/blog/a-fantastic-blog-post')7 ->metaCanonicalEnabled(true)8 ->metaRobots('🤖', '🤖', '🤖');
All of these are provided by the default methods and propagate through to the meta generator.
If you only want to render the meta generator, use seo()->generate('meta')
or @metadata('meta')
Twitter methods
The meta methods are provided by the Honeystone\Seo\Generators\TwitterGenerator
class.
Here's the full list:
1seo()2 ->twitterEnabled(true) //enabled by default, see config3 ->twitterSite('@MyWebsite')4 ->twitterCreator('@MyTwitter')5 ->twitterTitle('A fantastic blog post') //defaults to title()6 ->twitterDescription('Theres really a lot of great stuff in here...') //defaults to description()7 ->twitterImage('https://mywebsite.com/images/blog-1/cover-image.webp'); //defaults to the first in images()
You can learn more about this package, get full installation instructions, and view the source code on GitHub